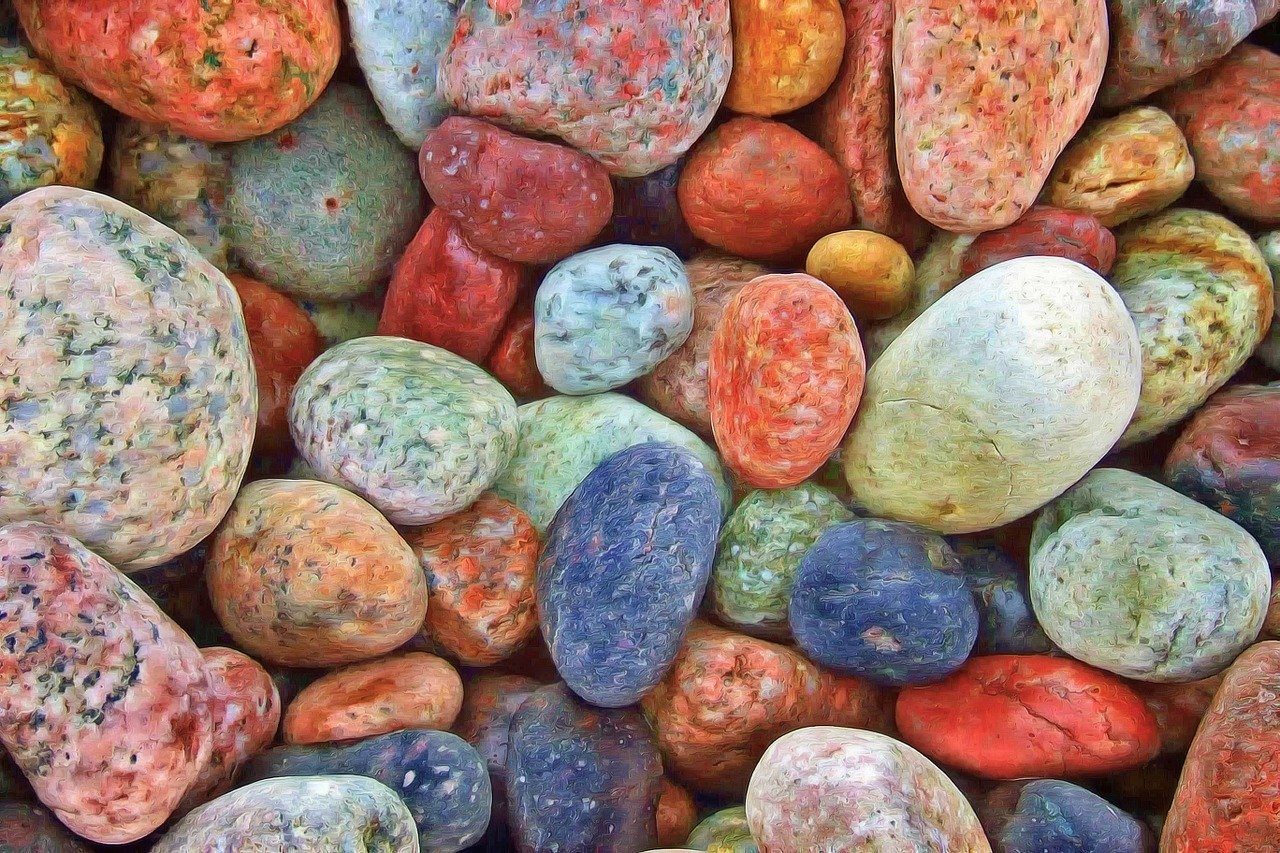
スポンサーリンク
※サイト運営にサーバーは必須です※
~このサイトもエックスサーバーを使用しています~
ソースコード
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class random1 : MonoBehaviour { public Text text; // Use this for initialization void Start () { text.text = ""; //Unity独自の方法。using UnityEngine; //0~10のランダムな実数(float) text.text += Random.Range(0f, 10f); text.text += "\r\n"; //0~10のランダムな整数。(int) text.text += Random.Range(0, 11); //10~11に入った実数を切り捨てして10とカウントしているため text.text += "\r\n"; //0~1.0の実数(float) text.text += Random.value; text.text += "\r\n"; //C#標準(?)の方法。using System; System.Random rnd = new System.Random(System.Environment.TickCount); text.text += rnd.Next(0, 11); //最小値が0の場合、rnd.Next(11)だけでもOK。(int) text.text += "\r\n"; text.text += rnd.NextDouble(); //0から1.0の実数(double) text.text += "\r\n"; } // Update is called once per frame void Update () { } } |
Unity独自の方法を使うか、C#の標準(?)的な方法を使うか、の2通りがある。
Unityで特に理由がなければ、Next()でなく、Random.Range()を使えばいいと思う。
※両方とも使ったことがあるが、正直に言って、どちらの方法もなんだかんだで乱数の偏りがある気がする。
※結果は以下のような感じになる。
※UIのTextに表示するようにInspecor上で設定しているため、上のソースコードをそのままコピペしても以下の結果は得られない。
あと、注意すべきポイントとして、using System;とRandom.Range()を同時に使うと以下のようなエラーが生じる
‘Random’は、’UnityEngine.Random’と’System.Random’間のあいまいな参照です。
このエラーの解釈は難しくない。
Randomが「UnityEngine.Random」の「Random」か「System.Random」の「Random」かはっきりせず困る。ただそれだけ。
これを解消したい場合は、Random.Range()をUnityEngine.Random.Range()と書き換えれば問題ない
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using System;//追加 public class random2 : MonoBehaviour { public Text text; // Use this for initialization void Start() { text.text = ""; //Unity独自の方法。using UnityEngine; text.text += UnityEngine.Random.Range(0f, 10f);//0~10のランダムな実数(float) text.text += "\r\n"; text.text += UnityEngine.Random.Range(0, 11);//0~10のランダムな整数。(int) //10~11に入った実数を切り捨てして10とカウントしているため text.text += "\r\n"; text.text += UnityEngine.Random.value;//0~1.0の実数(float) text.text += "\r\n"; //C#標準(?)の方法。using System; System.Random rnd = new System.Random(System.Environment.TickCount); text.text += rnd.Next(0, 11); //最小値が0の場合、rnd.Next(11)だけでもOK。(int) text.text += "\r\n"; text.text += rnd.NextDouble();//0から1.0の実数(double) text.text += "\r\n"; } // Update is called once per frame void Update() { } } |
関連記事
~プログラミングを勉強してみませんか?~
TechAcademy [テックアカデミー] は無料の体験講座が用意されているので、気軽に体験できます。
※私(サイト主)も無料体験講座を実際に受けてみました(→感想)